Have you ever used a phone app or computer program to add a silly “filter” to a photo?
When you use a filter, you are applying special image processing algorithms to the photo.
Loop-de-loops
These algorithms have to loop through every little speck of the photo and modify that speck (called a pixel) in some way (usually, changing its color).
To do this, most algorithms scan through the image, looking at every pixel in the first row, then looking through every pixel of the next row, continuing on until the end of the image is reached.
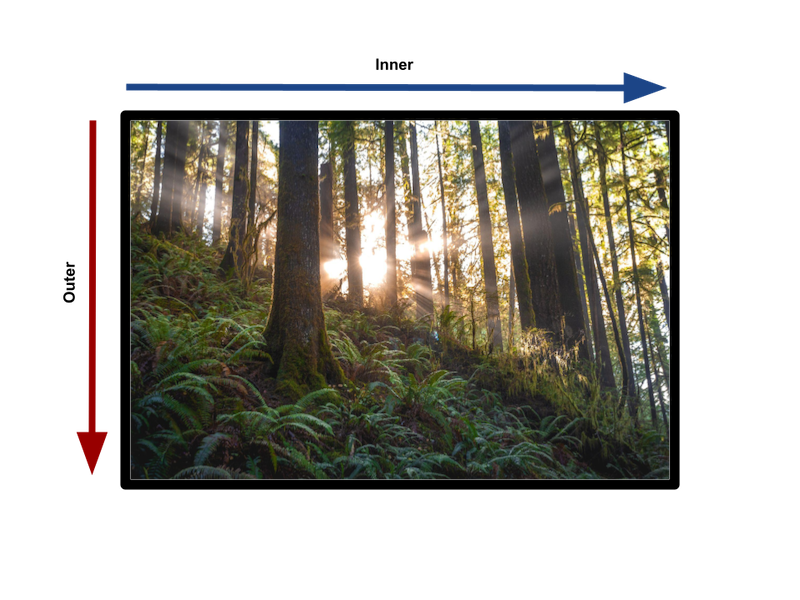
A computer would scan through this image by looking at every pixel in each row, starting with the first row and reading left to right. The “inner loop” goes through each pixel within a row, and the “outer loop” moves down to the next row once the first row is finished.
Rewired Reading
This is very similar to the way we read a page of a book. Our eyes start at the top left of the page, then we read each word of the first row, then each word of the second row, and so on.
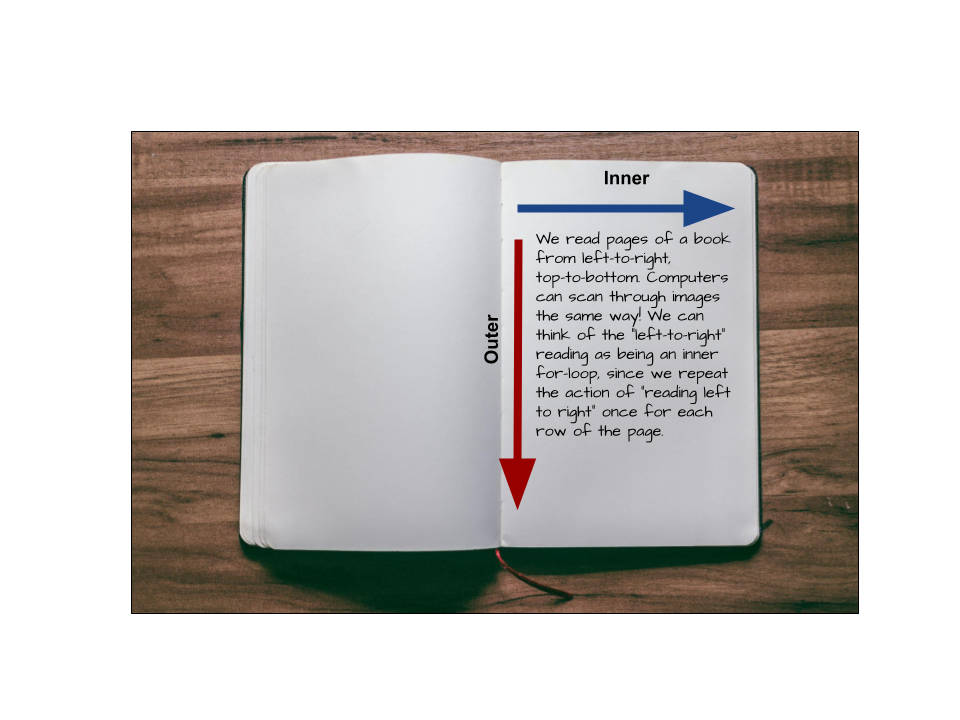
If we were to describe this reading algorithm we use, we might describe it like this:
for each line on the page,
for each word on the line,
read the word
Imagine thinking like a computer: we look at the first line, then, for each word on that line, we read the word. Once we hit the end of the line, our inner for-loop is done, and we move to the next line as instructed by our outer for-loop. Once we’ve read the last line on the page, our outer for-loop is done, and the algorithm is complete. (By the way, when we write instructions like a program, but without using a programming language, we call these instructions pseudocode. Pseudo means fake. In a way, this is fake code, since it isn’t written for a computer—it’s written for people.)
Just like reading, we can scan and apply a filter to our image in a similar way: for each row of the image, for each pixel in the row, apply our filter to the pixel
When we have two for-loops, with one happening inside the routine of the other, we call this a nested forloop algorithm.
Where else might we use a nested for loop?
Like My New Outfit?
Imagine you go shopping for a new outfit. You find six shirts and eight pairs of pants that you really like, but you aren’t sure which complete outfit (shirt + pants) you like best. Can we design an algorithm to try on all the possible outfits?
We know we want to try on all shirts, which will require one for loop.
We also want to try on all pants, which would take another for loop.
To make sure we try each combination, we must nest these for loops, like this: for each shirt, for each pair of pants, try on the outfit (shirt + pants)
We can illustrate the process like this:
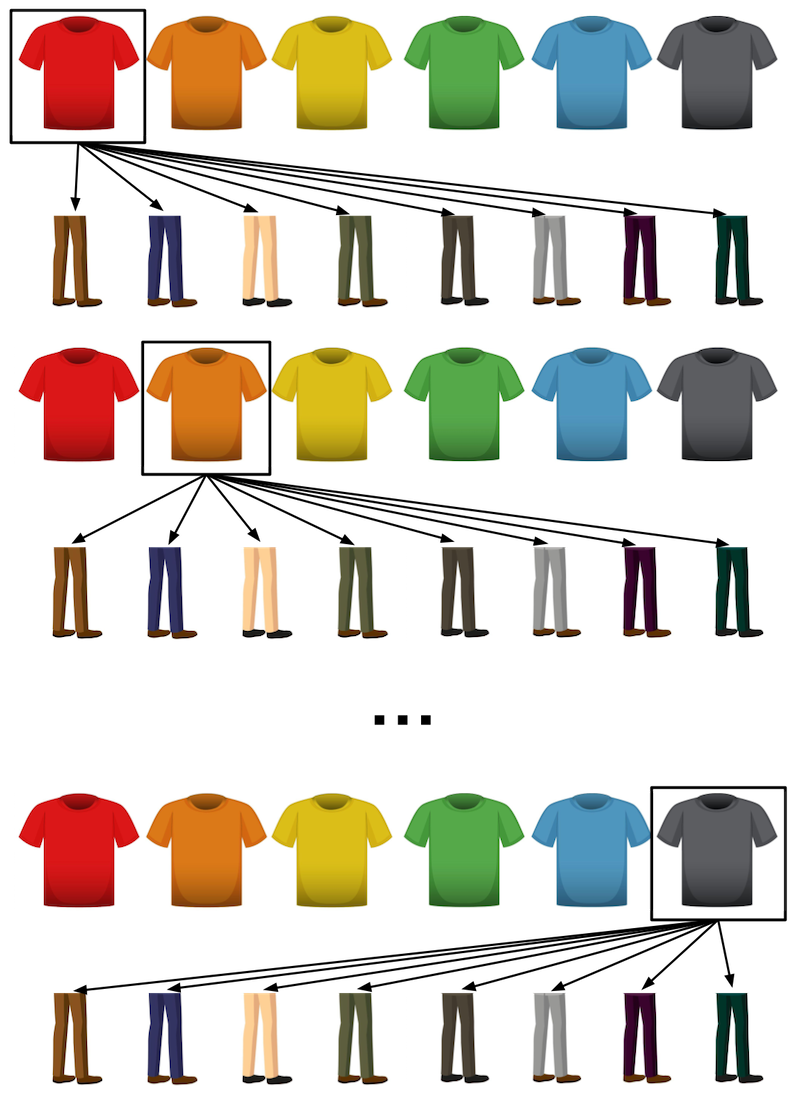
The outer loop tells us that we start by trying on the red shirt, then, the inner loop tells us to cycle through all possible pants. After the first shirt, the outer loop moves to the second shirt, then the inner loop repeats trying on all pants. Eventually, the outer loop reaches the final shirt, and the inner loop cycles through the pants one final time.
Can you think of other routines that use a nested for-loop pattern?
Try to think like a computer, and write your routine using pseudocode.
As a special challenge, what if we extend our examples above?
Instead of reading just a page, we would like to read an entire book, and instead of trying on outfits consisting of shirts and pants, we’d like to try on outfits of shirts, pants, and shoes.
Can you write pseudocode to describe these activities?
Check out the next issue’s article for the solution.
Learn More
Loops, A Fairy Tale
https://kidscodecs.com/loops-a-fairy-tale/
Algorithm Design
https://kidscodecs.com/algorithm-design/
Python For Loops
https://www.w3schools.com/python/python_for_loops.asp
For Loop
https://en.wikipedia.org/wiki/For_loop
Nested For Loops
https://runestone.academy/ns/books/published/csawesome/Unit4-Iteration/topic-4-4-nested-loops.html
Looping in Programming Languages
https://www.geeksforgeeks.org/scala-loopswhile-do-while-for-nested-loops/