The Raspberry Pi Pico is an excellent and affordable little device suitable for a wide range of electronics and projects. We’ve looked at it before in our “Getting Started with Raspberry Pi Pico” article where we talked about installing Thonny and programming the Pico using Micropython.
A popular use for many microcontrollers is to log some sort of data over a period of time. This can be useful in all kinds of microcontroller experiments. From monitoring the water level of a pond, the temperature of a room, the voltage of a battery system, or anything else that you can imagine.
While lots of microcontrollers are suitable for data logging, the Pico is a good choice for a few different reasons. First, it’s pretty cheap. If you need to make a few data logging platforms or you need to leave your data logger in a risky place, you want it to be an affordable unit.
Secondly, it has a reasonable amount of memory built-in. With its 2MB of flash memory, you can log a lot of information on it before you need additional memory. This, again, makes it more affordable but also more compact and less complex to set up.
With all that said, let’s look at how to set up a simple data-logging project using Micropython on the Pico. We’re going to create a simple temperature logging experiment that uses a common and affordable temperature sensor, a DS1820, to sense the temperature, and then we’ll log the temperature at regular intervals to a file on the Pico.
We’ve supplied the code example (A) and there are comments in the code to help make it more understandable, but let’s look at a few sections of the code to learn about what’s going on.
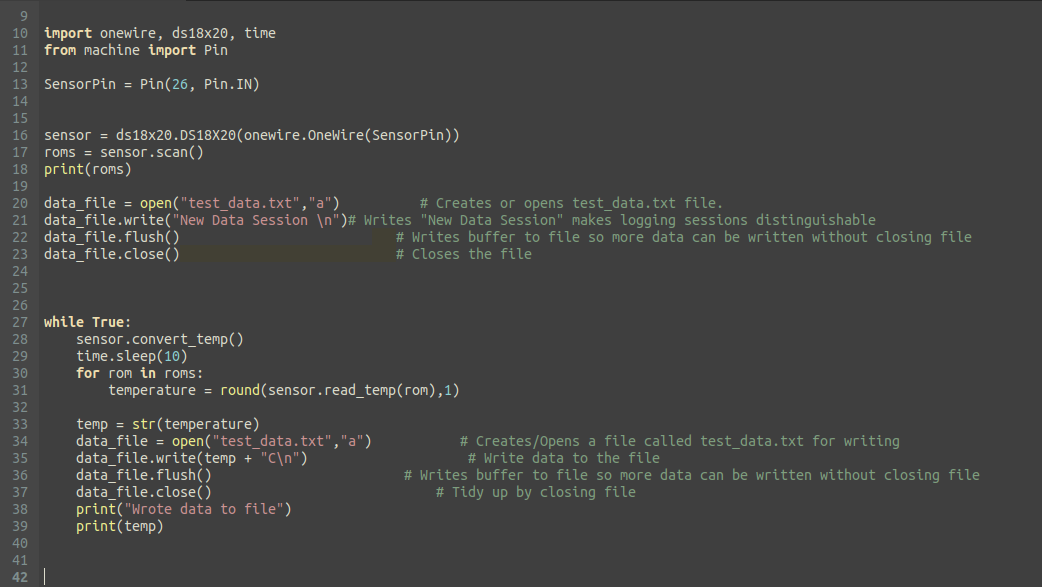
import onewire, ds18x20, time
from machine import Pin
In the first two lines above we use “import” or “from” and then “import” to import various libraries and parts of libraries we will need to reference in the main body of the code. The DS1820 sensor we are using is an example of a “onewire” type of sensor. Onewire or 1-wire is a type of bus that allows single sensors or networks of sensors to be connected to a microcontroller using only one conductor or one wire. This is really handy for simplifying wiring and for minimizing the amount of pins used to connect to sensors.
In our example, we are going to wire the positive (red) and negative (black) wires from the DS1820 to the 3v3 and GND pins and we are going to wire the DS1820 data wire (yellow) to pin 26 on the Pico. We are also going to wire a 10K resistor between the data line on pin 26 and the 3V3 pin to act as a pull-up resistor.
SensorPin = Pin(26, Pin.IN)
sensor = ds18x20.
DS18X20(onewire.
OneWire(SensorPin))
roms = sensor.scan()
print(roms)
These next four lines essentially set up the ds1820 sensor. We declare our pin connections and initialize the sensor.
data_file = open(“test_data.txt”,”a”)
data_file .write(” New Data Session\n “)
data_file.flush()
data_file.close()
The next four lines create and initialize a new text file saved in the root directory of the Pico. The first line data_file = open(“test_data. txt”,”a”) creates a file (if it doesn’t already exist) and you give it a name in quotes inside the brackets; we went with “test_data.txt”. We add the “a” which means that the file is appendable, meaning that if the file already exists from a previous logging session, the file will be opened and we will append or add new data in new lines under the existing text. As an aside, you can also use the letters “w” for write-only and “r” to open a file in read-only mode.
The next line “data_file.write(” New Data Session \n”)” uses the “write” command to write a line of data into the test_data.txt file we just created or reopened. Notice that what we write is contained in brackets and quotes and is a string. After the text, we add a “\n” in the string which creates a new line in the text file. This means that if we have made a previous log in the test_data.txt file, then this title “New Data Session” helps us keep track of each logging session.
The next line “data_file.flush()” ensures that any data in the buffer is written to the file and then clears the buffer. Finally for this section “data_file.close()” closes the file.
while True:
sensor.convert_temp()
time.sleep(10)
for rom in roms:
temperature = round(sensor.read_temp(rom),1) (B)
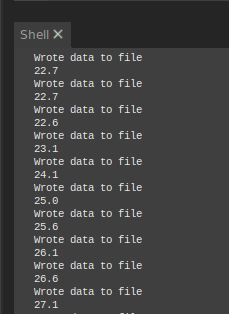
The next section of code sets up a loop and defines and structures the temperature sample we are going to take. Notice that the line reading “time.sleep(10)” sets the logging interval, so in this case we are going to run through this loop and log the temperature every 10 seconds. You can change this to whatever frequency you would like to log at.
temp = str(temperature)
data_file = open(“test_data.txt”,”a”)
data_file.write(temp + “C\n”)
data_file.flush()
data_file.close()
print(“Wrote data to file”)
print(temp)
The final 7 lines of the example begin with the line “temp=str(temperature)”. This simply converts “temperature” into a string object called “temp”. This is important as we can only write strings to our text file and if we tried to directly write “temperature” our code wouldn’t work. The next four lines should look familiar. We again re-open the test_data.txt file in append mode. We then write the string temp followed by “C” for Celsius and then add a new line break. We, again, flush and then close the file.
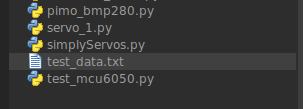
Finally, to help us check that the code is working we add a couple of “print” commands. If we run the code on the Pico from Thonny it will print the confirmation “Wrote data to file,” followed on a new line by the temperature value. This means that we can check our data logger is working before perhaps deploying it to run off an external power supply for a longer logging session. (C)
Once you have run the code successfully, when you next connect your Pico to Thonny you should see in the list of files on the Pico a text file named “test_data.txt”. You can click it and open it in a new tab in Thonny which is handy for a quick check of what it looks like. (D)
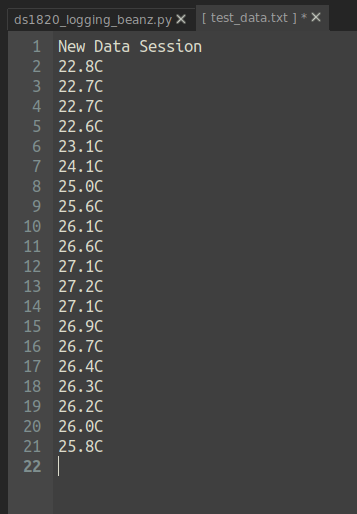
After opening it in a new Thonny tab, you can use the Thonny “Save As” icon to save the file over to your computer. We then used the free and open-source Libre Office LibreCalc application and imported it using just the default settings. We were rewarded with each temperature value in a separate spreadsheet cell. (E)
It’s really straightforward, then, to create graphs and charts of your data, amazing your friends, family, and perhaps teachers with your science and data skills.
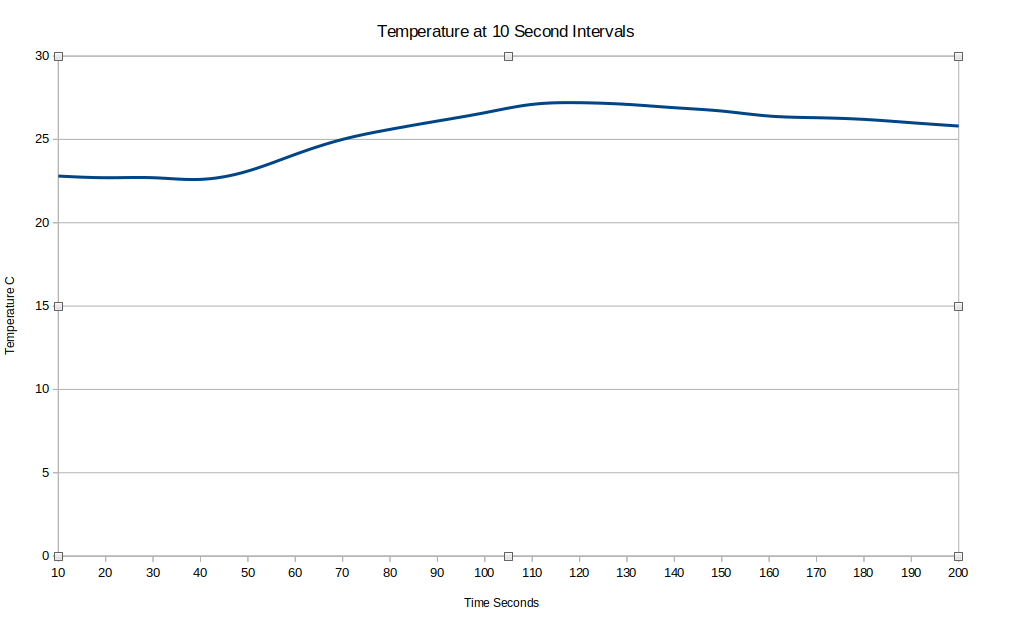
Learn More
What is Data Logging?
https://www.crowdstrike.com/cybersecurity-101/observability/data-logging/
Data Logging
https://www.ralstoninst.com/applications/what-is-data-logging
Data Logging for the Classroom
https://www.twinkl.com/teaching-wiki/data-logger
Data Logging in the Primary Classroom
https://www.technologytoteach.co.uk/data-logging-in-the-primary-classroom
Fun Ways for Kids to Generate Data
https://www.edutopia.org/article/fun-ways-get-kids-generate-and-manipulate-their-own-data/
Data Logging with Raspberry Pi Pico
https://www.instructables.com/Data-Logging-With-Raspberry-Pi-Pico/
Logging Sensor Data with Raspberry Pi Pico
https://halvorsen.blog/documents/technology/iot/pico/pico_datalogging.php